Fetching app lists, adding/removing apps from a list
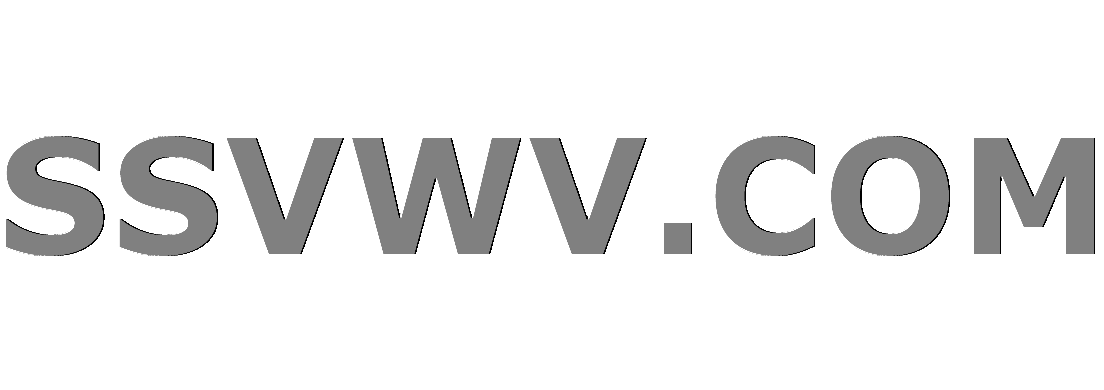
Multi tool use
up vote
0
down vote
favorite
I wrote a code that should help me fetch app lists from my database (set by different tables by their category - Microsoft Office apps, Other apps, System apps, ect').
I will add my class structure, and would like a review mainly for the architecture.
I'm not sure if I'm using the interface properly, if I even should use it like this. and if my way of extending the main class was done properly.
Firstly, I defined an interface:
Interface AppList {
// For getting lists from the database
public function getList();
// For Adding a new app to the list
public function addToList();
// For removing an app from the list
public function removeFromList();
}
Then I implement in a main class named "AppList" which is my main list class:
class Static_list implements AppList {
protected $db;
protected $table;
public function __construct() {
// get db instance
$this->db = Database::getInstance();
}
/**
*
* Gets the list by (table) name
* @param $table String Gets a name of a table (list)
* @throws Array/Boolian Returns an array of apps(/rows)
*
*/
public function getList() {
// SELECT * FROM $this->table
}
/**
*
* Adds a new app to the app lits - by (table) name
* @param $table String Gets a name of a table (list)
* @param $contents Array Gets an array of contents to apply in input.
* @throws Boolian Returns "ture" if successful, "false" if not (for mainly )
*
*/
public function addToList() {
// INSERT INTO $this->table ( field1, field2,...fieldN ) VALUES ( $contents[1], $contents[2],...$contents[N] );
}
/**
*
* Removes a new app to the app lits - by (table) name
* @param $table String Gets a name of a table (list)
* @param $id Init App ID to remove
* @throws Boolian Returns "ture" if successful, "false" if not (for mainly )
*
*/
public function removeFromList($id) {
// DELETE FROM $this->table WHERE id = $id;
}
}
As mentioned before, each list has a different table ('ms_app_list' db table, 'other_app_list' db table, 'system_app_list' db table, ect')
So for each table I'm going to have a different class that extends the main "AppList" class.
class Ms_app_list extends Static_list {
private $table = 'ms_app_list';
}
class Other_app_list extends Static_list {
private $table = 'other_app_list';
}
class System_app_list extends Static_list {
private $table = 'system_app_list';
}
So then if I want to print_r()
my "system apps list", I just:
$sys_apps = new System_app_list();
print_r($sys_apps->getList());
Is the interface not relevant for my case? At the beginning I was thinking this was irrelevant since I use them in the main class(Static_list
) and ill work without the interface too, but isn't this considered a "good practice" for code where more than one developer works on?
Should every list be a separate class that implements the interface, and extending a main class is a bad practice?
Example for only one list:
class Ms_app_list implements AppList {
private $table = 'ms_app_list';
private $db;
public function __construct() {
// get db instance
$this->db = Database::getInstance();
}
public function getList() {
// SELECT * FROM $this->table
}
public function addToList() {
// INSERT INTO $this->table ( field1, field2,...fieldN ) VALUES ( $contents[1], $contents[2],...$contents[N] );
}
public function removeFromList($id) {
// DELETE FROM $this->table WHERE id = $id;
}
}
I decided to use one main class (Static_list
) with the 3 "must included methods" and extend the class to other 3 lists classes to prevent code repetition.
php object-oriented
add a comment |
up vote
0
down vote
favorite
I wrote a code that should help me fetch app lists from my database (set by different tables by their category - Microsoft Office apps, Other apps, System apps, ect').
I will add my class structure, and would like a review mainly for the architecture.
I'm not sure if I'm using the interface properly, if I even should use it like this. and if my way of extending the main class was done properly.
Firstly, I defined an interface:
Interface AppList {
// For getting lists from the database
public function getList();
// For Adding a new app to the list
public function addToList();
// For removing an app from the list
public function removeFromList();
}
Then I implement in a main class named "AppList" which is my main list class:
class Static_list implements AppList {
protected $db;
protected $table;
public function __construct() {
// get db instance
$this->db = Database::getInstance();
}
/**
*
* Gets the list by (table) name
* @param $table String Gets a name of a table (list)
* @throws Array/Boolian Returns an array of apps(/rows)
*
*/
public function getList() {
// SELECT * FROM $this->table
}
/**
*
* Adds a new app to the app lits - by (table) name
* @param $table String Gets a name of a table (list)
* @param $contents Array Gets an array of contents to apply in input.
* @throws Boolian Returns "ture" if successful, "false" if not (for mainly )
*
*/
public function addToList() {
// INSERT INTO $this->table ( field1, field2,...fieldN ) VALUES ( $contents[1], $contents[2],...$contents[N] );
}
/**
*
* Removes a new app to the app lits - by (table) name
* @param $table String Gets a name of a table (list)
* @param $id Init App ID to remove
* @throws Boolian Returns "ture" if successful, "false" if not (for mainly )
*
*/
public function removeFromList($id) {
// DELETE FROM $this->table WHERE id = $id;
}
}
As mentioned before, each list has a different table ('ms_app_list' db table, 'other_app_list' db table, 'system_app_list' db table, ect')
So for each table I'm going to have a different class that extends the main "AppList" class.
class Ms_app_list extends Static_list {
private $table = 'ms_app_list';
}
class Other_app_list extends Static_list {
private $table = 'other_app_list';
}
class System_app_list extends Static_list {
private $table = 'system_app_list';
}
So then if I want to print_r()
my "system apps list", I just:
$sys_apps = new System_app_list();
print_r($sys_apps->getList());
Is the interface not relevant for my case? At the beginning I was thinking this was irrelevant since I use them in the main class(Static_list
) and ill work without the interface too, but isn't this considered a "good practice" for code where more than one developer works on?
Should every list be a separate class that implements the interface, and extending a main class is a bad practice?
Example for only one list:
class Ms_app_list implements AppList {
private $table = 'ms_app_list';
private $db;
public function __construct() {
// get db instance
$this->db = Database::getInstance();
}
public function getList() {
// SELECT * FROM $this->table
}
public function addToList() {
// INSERT INTO $this->table ( field1, field2,...fieldN ) VALUES ( $contents[1], $contents[2],...$contents[N] );
}
public function removeFromList($id) {
// DELETE FROM $this->table WHERE id = $id;
}
}
I decided to use one main class (Static_list
) with the 3 "must included methods" and extend the class to other 3 lists classes to prevent code repetition.
php object-oriented
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I wrote a code that should help me fetch app lists from my database (set by different tables by their category - Microsoft Office apps, Other apps, System apps, ect').
I will add my class structure, and would like a review mainly for the architecture.
I'm not sure if I'm using the interface properly, if I even should use it like this. and if my way of extending the main class was done properly.
Firstly, I defined an interface:
Interface AppList {
// For getting lists from the database
public function getList();
// For Adding a new app to the list
public function addToList();
// For removing an app from the list
public function removeFromList();
}
Then I implement in a main class named "AppList" which is my main list class:
class Static_list implements AppList {
protected $db;
protected $table;
public function __construct() {
// get db instance
$this->db = Database::getInstance();
}
/**
*
* Gets the list by (table) name
* @param $table String Gets a name of a table (list)
* @throws Array/Boolian Returns an array of apps(/rows)
*
*/
public function getList() {
// SELECT * FROM $this->table
}
/**
*
* Adds a new app to the app lits - by (table) name
* @param $table String Gets a name of a table (list)
* @param $contents Array Gets an array of contents to apply in input.
* @throws Boolian Returns "ture" if successful, "false" if not (for mainly )
*
*/
public function addToList() {
// INSERT INTO $this->table ( field1, field2,...fieldN ) VALUES ( $contents[1], $contents[2],...$contents[N] );
}
/**
*
* Removes a new app to the app lits - by (table) name
* @param $table String Gets a name of a table (list)
* @param $id Init App ID to remove
* @throws Boolian Returns "ture" if successful, "false" if not (for mainly )
*
*/
public function removeFromList($id) {
// DELETE FROM $this->table WHERE id = $id;
}
}
As mentioned before, each list has a different table ('ms_app_list' db table, 'other_app_list' db table, 'system_app_list' db table, ect')
So for each table I'm going to have a different class that extends the main "AppList" class.
class Ms_app_list extends Static_list {
private $table = 'ms_app_list';
}
class Other_app_list extends Static_list {
private $table = 'other_app_list';
}
class System_app_list extends Static_list {
private $table = 'system_app_list';
}
So then if I want to print_r()
my "system apps list", I just:
$sys_apps = new System_app_list();
print_r($sys_apps->getList());
Is the interface not relevant for my case? At the beginning I was thinking this was irrelevant since I use them in the main class(Static_list
) and ill work without the interface too, but isn't this considered a "good practice" for code where more than one developer works on?
Should every list be a separate class that implements the interface, and extending a main class is a bad practice?
Example for only one list:
class Ms_app_list implements AppList {
private $table = 'ms_app_list';
private $db;
public function __construct() {
// get db instance
$this->db = Database::getInstance();
}
public function getList() {
// SELECT * FROM $this->table
}
public function addToList() {
// INSERT INTO $this->table ( field1, field2,...fieldN ) VALUES ( $contents[1], $contents[2],...$contents[N] );
}
public function removeFromList($id) {
// DELETE FROM $this->table WHERE id = $id;
}
}
I decided to use one main class (Static_list
) with the 3 "must included methods" and extend the class to other 3 lists classes to prevent code repetition.
php object-oriented
I wrote a code that should help me fetch app lists from my database (set by different tables by their category - Microsoft Office apps, Other apps, System apps, ect').
I will add my class structure, and would like a review mainly for the architecture.
I'm not sure if I'm using the interface properly, if I even should use it like this. and if my way of extending the main class was done properly.
Firstly, I defined an interface:
Interface AppList {
// For getting lists from the database
public function getList();
// For Adding a new app to the list
public function addToList();
// For removing an app from the list
public function removeFromList();
}
Then I implement in a main class named "AppList" which is my main list class:
class Static_list implements AppList {
protected $db;
protected $table;
public function __construct() {
// get db instance
$this->db = Database::getInstance();
}
/**
*
* Gets the list by (table) name
* @param $table String Gets a name of a table (list)
* @throws Array/Boolian Returns an array of apps(/rows)
*
*/
public function getList() {
// SELECT * FROM $this->table
}
/**
*
* Adds a new app to the app lits - by (table) name
* @param $table String Gets a name of a table (list)
* @param $contents Array Gets an array of contents to apply in input.
* @throws Boolian Returns "ture" if successful, "false" if not (for mainly )
*
*/
public function addToList() {
// INSERT INTO $this->table ( field1, field2,...fieldN ) VALUES ( $contents[1], $contents[2],...$contents[N] );
}
/**
*
* Removes a new app to the app lits - by (table) name
* @param $table String Gets a name of a table (list)
* @param $id Init App ID to remove
* @throws Boolian Returns "ture" if successful, "false" if not (for mainly )
*
*/
public function removeFromList($id) {
// DELETE FROM $this->table WHERE id = $id;
}
}
As mentioned before, each list has a different table ('ms_app_list' db table, 'other_app_list' db table, 'system_app_list' db table, ect')
So for each table I'm going to have a different class that extends the main "AppList" class.
class Ms_app_list extends Static_list {
private $table = 'ms_app_list';
}
class Other_app_list extends Static_list {
private $table = 'other_app_list';
}
class System_app_list extends Static_list {
private $table = 'system_app_list';
}
So then if I want to print_r()
my "system apps list", I just:
$sys_apps = new System_app_list();
print_r($sys_apps->getList());
Is the interface not relevant for my case? At the beginning I was thinking this was irrelevant since I use them in the main class(Static_list
) and ill work without the interface too, but isn't this considered a "good practice" for code where more than one developer works on?
Should every list be a separate class that implements the interface, and extending a main class is a bad practice?
Example for only one list:
class Ms_app_list implements AppList {
private $table = 'ms_app_list';
private $db;
public function __construct() {
// get db instance
$this->db = Database::getInstance();
}
public function getList() {
// SELECT * FROM $this->table
}
public function addToList() {
// INSERT INTO $this->table ( field1, field2,...fieldN ) VALUES ( $contents[1], $contents[2],...$contents[N] );
}
public function removeFromList($id) {
// DELETE FROM $this->table WHERE id = $id;
}
}
I decided to use one main class (Static_list
) with the 3 "must included methods" and extend the class to other 3 lists classes to prevent code repetition.
php object-oriented
php object-oriented
asked Nov 18 at 14:25


Kar19
1175
1175
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f207922%2ffetching-app-lists-adding-removing-apps-from-a-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5vF zgxJem43NgZKVU9Dx9M7v2qD1t4Kp,cgs1