Cannot pass argument through /proc//fd/0
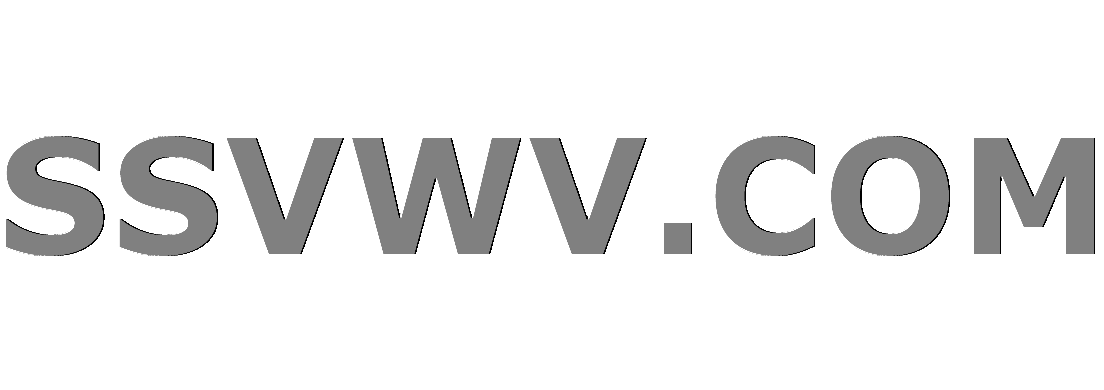
Multi tool use
I have written a basic C++ program as such:
#include <sstream>
#include <unistd.h>
#include <cstdio>
#include <iostream>
int main(void)
{
int foo;
std::cin >> foo;
FILE* fd = fopen("./output", "a+");
std::stringstream ss;
ss << getpid() << std::endl;
fputs(ss.str().c_str(), fd);
}
The code simply expects input from standard input, and appends its process id to an output file. After successful compilation, I execute the binary as background process ./my_binary&
, check its pid with jobs -l
, and try to pass a dummy integer to it either with printf "6" >/proc/<pid>/fd/0
or echo -e "6n" >/proc/<pid>/fd/0
. The process does not write in output file and exit as I expect it to. What am I doing wrong here? I can see the 0
descriptor in the process' proc directory but does it get somehow blocked when the process it thrown in the background?
Note that in contrast, I can exhibit the expected behaviour by fg <n>
ing and manually entering an integer.
Edit: As per Kusalananda's comment, I have realized that a SIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty.
Edit: Failing to find a way to mitigate SIGTTINT
issue, I decided to use a named pipe instead of stdin
. The code has been changed so (also I switched to C, having realized I ended up using pure C anyway):
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <stdio.h>
int main(void)
{
char input[3];
read(7, input, 2);
int num = atoi(input);
char output[256];
memset(output, 0, 256);
sprintf(output, "%d : %dn", getpid(), num);
write(13, output, strlen(output));
return 0;
}
I created a pipe: mkfifo input_pipe
, and called the process as ./process 7<input_pipe 13>>output
, passing the arguments into input_pipe
.
process io-redirection signals file-descriptors
add a comment |
I have written a basic C++ program as such:
#include <sstream>
#include <unistd.h>
#include <cstdio>
#include <iostream>
int main(void)
{
int foo;
std::cin >> foo;
FILE* fd = fopen("./output", "a+");
std::stringstream ss;
ss << getpid() << std::endl;
fputs(ss.str().c_str(), fd);
}
The code simply expects input from standard input, and appends its process id to an output file. After successful compilation, I execute the binary as background process ./my_binary&
, check its pid with jobs -l
, and try to pass a dummy integer to it either with printf "6" >/proc/<pid>/fd/0
or echo -e "6n" >/proc/<pid>/fd/0
. The process does not write in output file and exit as I expect it to. What am I doing wrong here? I can see the 0
descriptor in the process' proc directory but does it get somehow blocked when the process it thrown in the background?
Note that in contrast, I can exhibit the expected behaviour by fg <n>
ing and manually entering an integer.
Edit: As per Kusalananda's comment, I have realized that a SIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty.
Edit: Failing to find a way to mitigate SIGTTINT
issue, I decided to use a named pipe instead of stdin
. The code has been changed so (also I switched to C, having realized I ended up using pure C anyway):
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <stdio.h>
int main(void)
{
char input[3];
read(7, input, 2);
int num = atoi(input);
char output[256];
memset(output, 0, 256);
sprintf(output, "%d : %dn", getpid(), num);
write(13, output, strlen(output));
return 0;
}
I created a pipe: mkfifo input_pipe
, and called the process as ./process 7<input_pipe 13>>output
, passing the arguments into input_pipe
.
process io-redirection signals file-descriptors
1
What is the status of the process after you try to write to it? Most programs that read from the terminal as its standard input enters a "stopped" state when put into the background, since it blocks on the read until a terminal is present. Try e.g.( read thing ) &
in the shell.
– Kusalananda
Dec 12 at 12:00
Great, this has been a good starting point. The process is indeed being stopped, and it is also rejectingkill -SIGCONT <pid>
continue signals. I have looked further into it and seen that aSIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty. I'll see if I can trap and ignore this signal, or get input from some other file than stdin. I will keep here posted.
– corsel
Dec 12 at 13:09
2
If your intent is to have the process act like a daemon that can accept input from some unrelated process, the time-tested way of doing this is to have it read from a named pipe.
– Mark Plotnick
Dec 12 at 14:45
Thanks for the tip, I ended up doing that and edited the question accordingly. I have a few of these bckground jobs and once one pulls the input data out of the pipe, others starve. Do you know of any ways to deal with this more elegant than perhaps giving each process a different pipe, and copying the data to each of them, or appending n numbers of repeated input?
– corsel
Dec 12 at 15:01
add a comment |
I have written a basic C++ program as such:
#include <sstream>
#include <unistd.h>
#include <cstdio>
#include <iostream>
int main(void)
{
int foo;
std::cin >> foo;
FILE* fd = fopen("./output", "a+");
std::stringstream ss;
ss << getpid() << std::endl;
fputs(ss.str().c_str(), fd);
}
The code simply expects input from standard input, and appends its process id to an output file. After successful compilation, I execute the binary as background process ./my_binary&
, check its pid with jobs -l
, and try to pass a dummy integer to it either with printf "6" >/proc/<pid>/fd/0
or echo -e "6n" >/proc/<pid>/fd/0
. The process does not write in output file and exit as I expect it to. What am I doing wrong here? I can see the 0
descriptor in the process' proc directory but does it get somehow blocked when the process it thrown in the background?
Note that in contrast, I can exhibit the expected behaviour by fg <n>
ing and manually entering an integer.
Edit: As per Kusalananda's comment, I have realized that a SIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty.
Edit: Failing to find a way to mitigate SIGTTINT
issue, I decided to use a named pipe instead of stdin
. The code has been changed so (also I switched to C, having realized I ended up using pure C anyway):
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <stdio.h>
int main(void)
{
char input[3];
read(7, input, 2);
int num = atoi(input);
char output[256];
memset(output, 0, 256);
sprintf(output, "%d : %dn", getpid(), num);
write(13, output, strlen(output));
return 0;
}
I created a pipe: mkfifo input_pipe
, and called the process as ./process 7<input_pipe 13>>output
, passing the arguments into input_pipe
.
process io-redirection signals file-descriptors
I have written a basic C++ program as such:
#include <sstream>
#include <unistd.h>
#include <cstdio>
#include <iostream>
int main(void)
{
int foo;
std::cin >> foo;
FILE* fd = fopen("./output", "a+");
std::stringstream ss;
ss << getpid() << std::endl;
fputs(ss.str().c_str(), fd);
}
The code simply expects input from standard input, and appends its process id to an output file. After successful compilation, I execute the binary as background process ./my_binary&
, check its pid with jobs -l
, and try to pass a dummy integer to it either with printf "6" >/proc/<pid>/fd/0
or echo -e "6n" >/proc/<pid>/fd/0
. The process does not write in output file and exit as I expect it to. What am I doing wrong here? I can see the 0
descriptor in the process' proc directory but does it get somehow blocked when the process it thrown in the background?
Note that in contrast, I can exhibit the expected behaviour by fg <n>
ing and manually entering an integer.
Edit: As per Kusalananda's comment, I have realized that a SIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty.
Edit: Failing to find a way to mitigate SIGTTINT
issue, I decided to use a named pipe instead of stdin
. The code has been changed so (also I switched to C, having realized I ended up using pure C anyway):
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <stdio.h>
int main(void)
{
char input[3];
read(7, input, 2);
int num = atoi(input);
char output[256];
memset(output, 0, 256);
sprintf(output, "%d : %dn", getpid(), num);
write(13, output, strlen(output));
return 0;
}
I created a pipe: mkfifo input_pipe
, and called the process as ./process 7<input_pipe 13>>output
, passing the arguments into input_pipe
.
process io-redirection signals file-descriptors
process io-redirection signals file-descriptors
edited Dec 12 at 14:58
asked Dec 12 at 11:54


corsel
7519
7519
1
What is the status of the process after you try to write to it? Most programs that read from the terminal as its standard input enters a "stopped" state when put into the background, since it blocks on the read until a terminal is present. Try e.g.( read thing ) &
in the shell.
– Kusalananda
Dec 12 at 12:00
Great, this has been a good starting point. The process is indeed being stopped, and it is also rejectingkill -SIGCONT <pid>
continue signals. I have looked further into it and seen that aSIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty. I'll see if I can trap and ignore this signal, or get input from some other file than stdin. I will keep here posted.
– corsel
Dec 12 at 13:09
2
If your intent is to have the process act like a daemon that can accept input from some unrelated process, the time-tested way of doing this is to have it read from a named pipe.
– Mark Plotnick
Dec 12 at 14:45
Thanks for the tip, I ended up doing that and edited the question accordingly. I have a few of these bckground jobs and once one pulls the input data out of the pipe, others starve. Do you know of any ways to deal with this more elegant than perhaps giving each process a different pipe, and copying the data to each of them, or appending n numbers of repeated input?
– corsel
Dec 12 at 15:01
add a comment |
1
What is the status of the process after you try to write to it? Most programs that read from the terminal as its standard input enters a "stopped" state when put into the background, since it blocks on the read until a terminal is present. Try e.g.( read thing ) &
in the shell.
– Kusalananda
Dec 12 at 12:00
Great, this has been a good starting point. The process is indeed being stopped, and it is also rejectingkill -SIGCONT <pid>
continue signals. I have looked further into it and seen that aSIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty. I'll see if I can trap and ignore this signal, or get input from some other file than stdin. I will keep here posted.
– corsel
Dec 12 at 13:09
2
If your intent is to have the process act like a daemon that can accept input from some unrelated process, the time-tested way of doing this is to have it read from a named pipe.
– Mark Plotnick
Dec 12 at 14:45
Thanks for the tip, I ended up doing that and edited the question accordingly. I have a few of these bckground jobs and once one pulls the input data out of the pipe, others starve. Do you know of any ways to deal with this more elegant than perhaps giving each process a different pipe, and copying the data to each of them, or appending n numbers of repeated input?
– corsel
Dec 12 at 15:01
1
1
What is the status of the process after you try to write to it? Most programs that read from the terminal as its standard input enters a "stopped" state when put into the background, since it blocks on the read until a terminal is present. Try e.g.
( read thing ) &
in the shell.– Kusalananda
Dec 12 at 12:00
What is the status of the process after you try to write to it? Most programs that read from the terminal as its standard input enters a "stopped" state when put into the background, since it blocks on the read until a terminal is present. Try e.g.
( read thing ) &
in the shell.– Kusalananda
Dec 12 at 12:00
Great, this has been a good starting point. The process is indeed being stopped, and it is also rejecting
kill -SIGCONT <pid>
continue signals. I have looked further into it and seen that a SIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty. I'll see if I can trap and ignore this signal, or get input from some other file than stdin. I will keep here posted.– corsel
Dec 12 at 13:09
Great, this has been a good starting point. The process is indeed being stopped, and it is also rejecting
kill -SIGCONT <pid>
continue signals. I have looked further into it and seen that a SIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty. I'll see if I can trap and ignore this signal, or get input from some other file than stdin. I will keep here posted.– corsel
Dec 12 at 13:09
2
2
If your intent is to have the process act like a daemon that can accept input from some unrelated process, the time-tested way of doing this is to have it read from a named pipe.
– Mark Plotnick
Dec 12 at 14:45
If your intent is to have the process act like a daemon that can accept input from some unrelated process, the time-tested way of doing this is to have it read from a named pipe.
– Mark Plotnick
Dec 12 at 14:45
Thanks for the tip, I ended up doing that and edited the question accordingly. I have a few of these bckground jobs and once one pulls the input data out of the pipe, others starve. Do you know of any ways to deal with this more elegant than perhaps giving each process a different pipe, and copying the data to each of them, or appending n numbers of repeated input?
– corsel
Dec 12 at 15:01
Thanks for the tip, I ended up doing that and edited the question accordingly. I have a few of these bckground jobs and once one pulls the input data out of the pipe, others starve. Do you know of any ways to deal with this more elegant than perhaps giving each process a different pipe, and copying the data to each of them, or appending n numbers of repeated input?
– corsel
Dec 12 at 15:01
add a comment |
active
oldest
votes
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "106"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f487560%2fcannot-pass-argument-through-proc-pid-fd-0%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Unix & Linux Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f487560%2fcannot-pass-argument-through-proc-pid-fd-0%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
iQ,8ldsHCzBit7pD0geHjcOp16dTgte1xOmbX6zmHFZF5Gh,VsfXFE1Wef,Q7styswa1aYBCa,Aw
1
What is the status of the process after you try to write to it? Most programs that read from the terminal as its standard input enters a "stopped" state when put into the background, since it blocks on the read until a terminal is present. Try e.g.
( read thing ) &
in the shell.– Kusalananda
Dec 12 at 12:00
Great, this has been a good starting point. The process is indeed being stopped, and it is also rejecting
kill -SIGCONT <pid>
continue signals. I have looked further into it and seen that aSIGTTIN
signal is being emitted for processes which have been backgrounded while expecting input from tty. I'll see if I can trap and ignore this signal, or get input from some other file than stdin. I will keep here posted.– corsel
Dec 12 at 13:09
2
If your intent is to have the process act like a daemon that can accept input from some unrelated process, the time-tested way of doing this is to have it read from a named pipe.
– Mark Plotnick
Dec 12 at 14:45
Thanks for the tip, I ended up doing that and edited the question accordingly. I have a few of these bckground jobs and once one pulls the input data out of the pipe, others starve. Do you know of any ways to deal with this more elegant than perhaps giving each process a different pipe, and copying the data to each of them, or appending n numbers of repeated input?
– corsel
Dec 12 at 15:01