Solving square operational [on hold]
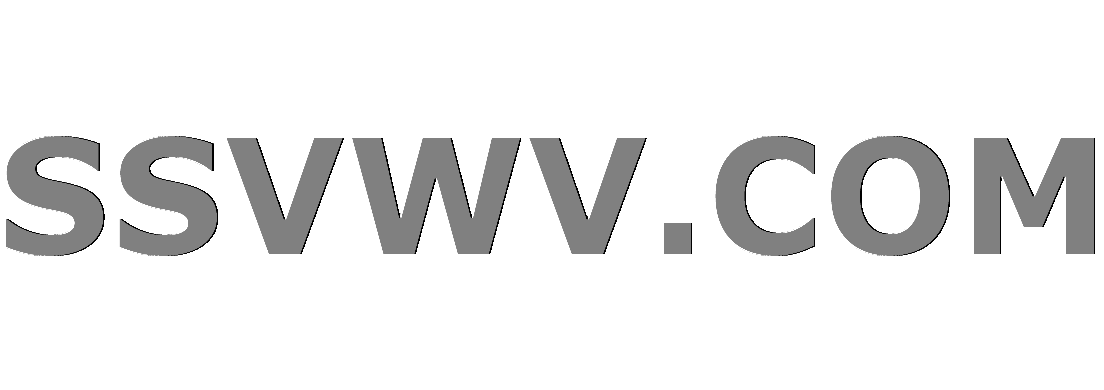
Multi tool use
up vote
-1
down vote
favorite
I tried to build simple code that solved square operational with value n
using python, plus, I want to learn recursion. I made three different style code like this:
First code
def pangkat(nilai, pangkat):
a = int(1)
for i in range(pangkat):
a = a * nilai
return a
if __name__ == "__main__":
print(pangkat(13, 8181))
Second Code
def pangkat(nilai, pangkat):
hasil = nilai**pangkat
return hasil
if __name__ == "__main__":
print(pangkat(13, 8181))
Third Code
def pangkat(nilai, pangkatnilai):
if pangkatnilai == 1:
return nilai
return nilai * pangkat(nilai, pangkatnilai-1)
if __name__ == "__main__":
print(pangkat(13,8181))
Note: param nilai
as number that will be raised, and pangkat
as number that will raised param nilai
), all of this code works well for example, when I fill the nilai
and param
.
Input 0
pangkat(13, 12)
Output 0
23298085122481
The problem occurred when I changed the param pangkat
>= 1000, it will give me an error but only in the third code.
Traceback (most recent call last):
File "pang3.py", line 8, in <module>
print(pangkat(13,1000))
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
[Previous line repeated 995 more times]
File "pang3.py", line 2, in pangkat
if pangkatnilai == 1:
RecursionError: maximum recursion depth exceeded in comparison
While the first and second code works well, what could go wrong with my recursion function? Plus, I need an explanation for it.
python python-3.x recursion
New contributor
Gagantous is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by Jamal♦ 4 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
up vote
-1
down vote
favorite
I tried to build simple code that solved square operational with value n
using python, plus, I want to learn recursion. I made three different style code like this:
First code
def pangkat(nilai, pangkat):
a = int(1)
for i in range(pangkat):
a = a * nilai
return a
if __name__ == "__main__":
print(pangkat(13, 8181))
Second Code
def pangkat(nilai, pangkat):
hasil = nilai**pangkat
return hasil
if __name__ == "__main__":
print(pangkat(13, 8181))
Third Code
def pangkat(nilai, pangkatnilai):
if pangkatnilai == 1:
return nilai
return nilai * pangkat(nilai, pangkatnilai-1)
if __name__ == "__main__":
print(pangkat(13,8181))
Note: param nilai
as number that will be raised, and pangkat
as number that will raised param nilai
), all of this code works well for example, when I fill the nilai
and param
.
Input 0
pangkat(13, 12)
Output 0
23298085122481
The problem occurred when I changed the param pangkat
>= 1000, it will give me an error but only in the third code.
Traceback (most recent call last):
File "pang3.py", line 8, in <module>
print(pangkat(13,1000))
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
[Previous line repeated 995 more times]
File "pang3.py", line 2, in pangkat
if pangkatnilai == 1:
RecursionError: maximum recursion depth exceeded in comparison
While the first and second code works well, what could go wrong with my recursion function? Plus, I need an explanation for it.
python python-3.x recursion
New contributor
Gagantous is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by Jamal♦ 4 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I tried to build simple code that solved square operational with value n
using python, plus, I want to learn recursion. I made three different style code like this:
First code
def pangkat(nilai, pangkat):
a = int(1)
for i in range(pangkat):
a = a * nilai
return a
if __name__ == "__main__":
print(pangkat(13, 8181))
Second Code
def pangkat(nilai, pangkat):
hasil = nilai**pangkat
return hasil
if __name__ == "__main__":
print(pangkat(13, 8181))
Third Code
def pangkat(nilai, pangkatnilai):
if pangkatnilai == 1:
return nilai
return nilai * pangkat(nilai, pangkatnilai-1)
if __name__ == "__main__":
print(pangkat(13,8181))
Note: param nilai
as number that will be raised, and pangkat
as number that will raised param nilai
), all of this code works well for example, when I fill the nilai
and param
.
Input 0
pangkat(13, 12)
Output 0
23298085122481
The problem occurred when I changed the param pangkat
>= 1000, it will give me an error but only in the third code.
Traceback (most recent call last):
File "pang3.py", line 8, in <module>
print(pangkat(13,1000))
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
[Previous line repeated 995 more times]
File "pang3.py", line 2, in pangkat
if pangkatnilai == 1:
RecursionError: maximum recursion depth exceeded in comparison
While the first and second code works well, what could go wrong with my recursion function? Plus, I need an explanation for it.
python python-3.x recursion
New contributor
Gagantous is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I tried to build simple code that solved square operational with value n
using python, plus, I want to learn recursion. I made three different style code like this:
First code
def pangkat(nilai, pangkat):
a = int(1)
for i in range(pangkat):
a = a * nilai
return a
if __name__ == "__main__":
print(pangkat(13, 8181))
Second Code
def pangkat(nilai, pangkat):
hasil = nilai**pangkat
return hasil
if __name__ == "__main__":
print(pangkat(13, 8181))
Third Code
def pangkat(nilai, pangkatnilai):
if pangkatnilai == 1:
return nilai
return nilai * pangkat(nilai, pangkatnilai-1)
if __name__ == "__main__":
print(pangkat(13,8181))
Note: param nilai
as number that will be raised, and pangkat
as number that will raised param nilai
), all of this code works well for example, when I fill the nilai
and param
.
Input 0
pangkat(13, 12)
Output 0
23298085122481
The problem occurred when I changed the param pangkat
>= 1000, it will give me an error but only in the third code.
Traceback (most recent call last):
File "pang3.py", line 8, in <module>
print(pangkat(13,1000))
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
File "pang3.py", line 5, in pangkat
return nilai * pangkat(nilai, pangkatnilai-1)
[Previous line repeated 995 more times]
File "pang3.py", line 2, in pangkat
if pangkatnilai == 1:
RecursionError: maximum recursion depth exceeded in comparison
While the first and second code works well, what could go wrong with my recursion function? Plus, I need an explanation for it.
python python-3.x recursion
python python-3.x recursion
New contributor
Gagantous is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Gagantous is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 4 hours ago


Jamal♦
30.2k11115226
30.2k11115226
New contributor
Gagantous is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 4 hours ago


Gagantous
992
992
New contributor
Gagantous is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Gagantous is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Gagantous is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by Jamal♦ 4 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
put on hold as off-topic by Jamal♦ 4 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
MY xvJXQnPDMZehMZ GYRMvfRxlXd,WUQ,fxflYro0