Simple Quiz game with Strategy and Factory pattern
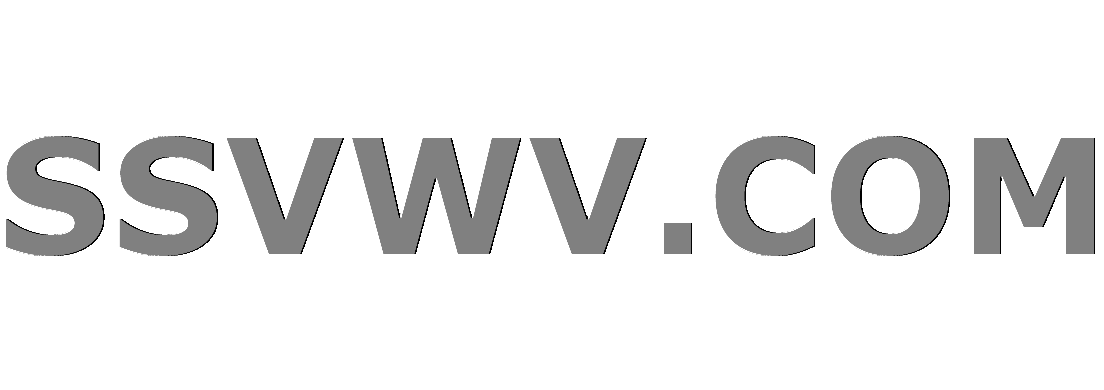
Multi tool use
I have a simple quiz game where user can give simple answers to simple questions and program will give a result.
Model classes looks like that:
public class Answer
{
public Answer(int id, int questionId, string content)
{
Id = id;
Content = content;
QuestionId = questionId;
}
public int Id { get; private set; }
public int QuestionId { get; private set; }
public string Content { get; private set; }
}
public class Question
{
public Question(int id, string content, char answer)
{
Id = id;
Content = content;
Answer = answer;
}
public int Id { get; private set; }
public string Content { get; private set; }
public char Answer { get; private set; }
}
Abstractions:
interface IAnswerService
{
List<IList<Answer>> GetAnswers();
}
interface IQuestionService
{
IList<Question> GetQuestions();
}
public interface ICalculationService
{
int CalculatePoints(Dictionary<Question, char> userAnswers);
}
Constants:
public static class Constants
{
public static class Answers
{
public const char A = 'a';
public const char B = 'b';
public const char C = 'c';
public const char D = 'd';
}
}
Core services:
static class Factory
{
public static T CreateInstance<T>() where T : new()
{
return new T();
}
}
Services:
public class AnswerService : IAnswerService
{
public List<IList<Answer>> GetAnswers()
{
return new List<IList<Answer>>() {
new List<Answer>() { new Answer(11, 3, "Sequoia"), new Answer(12, 3,
"Berch"), new Answer(13, 3, "Lindens"), new Answer(14, 3, "Alder") },
new List<Answer>() { new Answer(1, 1, "1"), new Answer(2, 1, "2"),
new Answer(3, 1, "5"), new Answer(4, 1, "6") },
new List<Answer>() { new Answer(7, 2, "More than 1"), new Answer(
8, 2, "More than 2"), new Answer(9, 2, "More than 5"),
new Answer(10, 2, "More than 6") },
new List<Answer>() { new Answer(15, 4, "yes, I do!"), new
Answer(16, 4, "Sure!"), new Answer(17, 4, "Exactly"), new
Answer(18, 4, "Yeap!") }
};
}
}
CalculationAnswerByAdding
class of Services:
class CalculationAnswerByAdding : ICalculationService
{
public int CalculatePoints(Dictionary<Question, char> userAnswers)
{
var sum = 0;
foreach (var question in userAnswers)
{
if (question.Key.Answer == question.Value)
sum += 1;
}
return sum;
}
}
CalculationAnswerBySubtracting
of Services:
class CalculationAnswerBySubtracting : ICalculationService
{
public int CalculatePoints(Dictionary<Question, char> userAnswers)
{
var sum = 10;
foreach (var question in userAnswers)
{
if (question.Key.Answer == question.Value)
sum -= 1;
}
return sum;
}
}
public class QuestionService : IQuestionService
{
public IList<Question> GetQuestions()
{
return new List<Question>() {
new Question(1, "How many are there contintents?",
Constants.Constants.Answers.A),
new Question(2, "How many are there colours?", Constants.Constants.Answers.B),
new Question(3, "What is the tallest tree?", Constants.Constants.Answers.C),
new Question(4, "Do you like dolphins?", Constants.Constants.Answers.D),
};
}
}
And Program.cs:
Main()
method of Program.cs
:
static void Main(string args)
{
IQuestionService questionService = Factory.CreateInstance<QuestionService>();
var questions = questionService.GetQuestions();
IAnswerService answerService = Factory.CreateInstance<AnswerService>();
var answers = answerService.GetAnswers();
var questionAnswers = questions.ToDictionary(q => q,
q => answers
.SelectMany(a => a)
.Where(b => b.QuestionId == q.Id)
.ToList());
var userAnswers = new Dictionary<Question, char>();
GetAsnwers(questionAnswers, userAnswers);
ICalculationService calculationService = Factory
.CreateInstance<CalculationAnswerByAdding>();
var userSum = calculationService.CalculatePoints(userAnswers);
Console.WriteLine(userSum > 3 ? $"Yeah, it is great. Your points are {userSum}."
: $"Hey, it is great. Your points are {userSum}");
}
GetAsnwers()
method of Program.cs
:
private static void GetAsnwers(Dictionary<Question, List<Answer>>
questionAnswers, Dictionary<Question, char> userAnswers )
{
foreach (var questionAnsw in questionAnswers)
{
AskQuestion(questionAnsw);
List<char> allowedAnswers = new List<char>()
{
Constants.Constants.Answers.A,
Constants.Constants.Answers.B,
Constants.Constants.Answers.C,
Constants.Constants.Answers.D,
};
while (true)
{
var userKey = Console.ReadKey().KeyChar;
Console.WriteLine();
if (!allowedAnswers.Contains(userKey))
{
AskQuestion(questionAnsw, true);
}
else
{
userAnswers.Add(questionAnsw.Key, userKey);
break;
}
}
}
}
AskQuestion()
method of Program.cs
:
private static void AskQuestion(KeyValuePair<Question, List<Answer>> questionAnswer,
bool showPossibleKeys = false)
{
if (showPossibleKeys) {
Console.WriteLine();
Console.WriteLine("Possible keys are A, B, C or D");
}
Console.WriteLine(questionAnswer.Key.Content);
questionAnswer.Value
.ForEach(a => Console.WriteLine(a.Content));
}
Guys, please, see my code. Is my code solid? Do I correctly use strategy and factory patterns? Any improvements and comments are greatly appreciated.
In addition, do my classes organized well? Especially, is it okay that I moved Factory
into folder CoreServices
? Or is there a better way to organize classes?
c#
add a comment |
I have a simple quiz game where user can give simple answers to simple questions and program will give a result.
Model classes looks like that:
public class Answer
{
public Answer(int id, int questionId, string content)
{
Id = id;
Content = content;
QuestionId = questionId;
}
public int Id { get; private set; }
public int QuestionId { get; private set; }
public string Content { get; private set; }
}
public class Question
{
public Question(int id, string content, char answer)
{
Id = id;
Content = content;
Answer = answer;
}
public int Id { get; private set; }
public string Content { get; private set; }
public char Answer { get; private set; }
}
Abstractions:
interface IAnswerService
{
List<IList<Answer>> GetAnswers();
}
interface IQuestionService
{
IList<Question> GetQuestions();
}
public interface ICalculationService
{
int CalculatePoints(Dictionary<Question, char> userAnswers);
}
Constants:
public static class Constants
{
public static class Answers
{
public const char A = 'a';
public const char B = 'b';
public const char C = 'c';
public const char D = 'd';
}
}
Core services:
static class Factory
{
public static T CreateInstance<T>() where T : new()
{
return new T();
}
}
Services:
public class AnswerService : IAnswerService
{
public List<IList<Answer>> GetAnswers()
{
return new List<IList<Answer>>() {
new List<Answer>() { new Answer(11, 3, "Sequoia"), new Answer(12, 3,
"Berch"), new Answer(13, 3, "Lindens"), new Answer(14, 3, "Alder") },
new List<Answer>() { new Answer(1, 1, "1"), new Answer(2, 1, "2"),
new Answer(3, 1, "5"), new Answer(4, 1, "6") },
new List<Answer>() { new Answer(7, 2, "More than 1"), new Answer(
8, 2, "More than 2"), new Answer(9, 2, "More than 5"),
new Answer(10, 2, "More than 6") },
new List<Answer>() { new Answer(15, 4, "yes, I do!"), new
Answer(16, 4, "Sure!"), new Answer(17, 4, "Exactly"), new
Answer(18, 4, "Yeap!") }
};
}
}
CalculationAnswerByAdding
class of Services:
class CalculationAnswerByAdding : ICalculationService
{
public int CalculatePoints(Dictionary<Question, char> userAnswers)
{
var sum = 0;
foreach (var question in userAnswers)
{
if (question.Key.Answer == question.Value)
sum += 1;
}
return sum;
}
}
CalculationAnswerBySubtracting
of Services:
class CalculationAnswerBySubtracting : ICalculationService
{
public int CalculatePoints(Dictionary<Question, char> userAnswers)
{
var sum = 10;
foreach (var question in userAnswers)
{
if (question.Key.Answer == question.Value)
sum -= 1;
}
return sum;
}
}
public class QuestionService : IQuestionService
{
public IList<Question> GetQuestions()
{
return new List<Question>() {
new Question(1, "How many are there contintents?",
Constants.Constants.Answers.A),
new Question(2, "How many are there colours?", Constants.Constants.Answers.B),
new Question(3, "What is the tallest tree?", Constants.Constants.Answers.C),
new Question(4, "Do you like dolphins?", Constants.Constants.Answers.D),
};
}
}
And Program.cs:
Main()
method of Program.cs
:
static void Main(string args)
{
IQuestionService questionService = Factory.CreateInstance<QuestionService>();
var questions = questionService.GetQuestions();
IAnswerService answerService = Factory.CreateInstance<AnswerService>();
var answers = answerService.GetAnswers();
var questionAnswers = questions.ToDictionary(q => q,
q => answers
.SelectMany(a => a)
.Where(b => b.QuestionId == q.Id)
.ToList());
var userAnswers = new Dictionary<Question, char>();
GetAsnwers(questionAnswers, userAnswers);
ICalculationService calculationService = Factory
.CreateInstance<CalculationAnswerByAdding>();
var userSum = calculationService.CalculatePoints(userAnswers);
Console.WriteLine(userSum > 3 ? $"Yeah, it is great. Your points are {userSum}."
: $"Hey, it is great. Your points are {userSum}");
}
GetAsnwers()
method of Program.cs
:
private static void GetAsnwers(Dictionary<Question, List<Answer>>
questionAnswers, Dictionary<Question, char> userAnswers )
{
foreach (var questionAnsw in questionAnswers)
{
AskQuestion(questionAnsw);
List<char> allowedAnswers = new List<char>()
{
Constants.Constants.Answers.A,
Constants.Constants.Answers.B,
Constants.Constants.Answers.C,
Constants.Constants.Answers.D,
};
while (true)
{
var userKey = Console.ReadKey().KeyChar;
Console.WriteLine();
if (!allowedAnswers.Contains(userKey))
{
AskQuestion(questionAnsw, true);
}
else
{
userAnswers.Add(questionAnsw.Key, userKey);
break;
}
}
}
}
AskQuestion()
method of Program.cs
:
private static void AskQuestion(KeyValuePair<Question, List<Answer>> questionAnswer,
bool showPossibleKeys = false)
{
if (showPossibleKeys) {
Console.WriteLine();
Console.WriteLine("Possible keys are A, B, C or D");
}
Console.WriteLine(questionAnswer.Key.Content);
questionAnswer.Value
.ForEach(a => Console.WriteLine(a.Content));
}
Guys, please, see my code. Is my code solid? Do I correctly use strategy and factory patterns? Any improvements and comments are greatly appreciated.
In addition, do my classes organized well? Especially, is it okay that I moved Factory
into folder CoreServices
? Or is there a better way to organize classes?
c#
add a comment |
I have a simple quiz game where user can give simple answers to simple questions and program will give a result.
Model classes looks like that:
public class Answer
{
public Answer(int id, int questionId, string content)
{
Id = id;
Content = content;
QuestionId = questionId;
}
public int Id { get; private set; }
public int QuestionId { get; private set; }
public string Content { get; private set; }
}
public class Question
{
public Question(int id, string content, char answer)
{
Id = id;
Content = content;
Answer = answer;
}
public int Id { get; private set; }
public string Content { get; private set; }
public char Answer { get; private set; }
}
Abstractions:
interface IAnswerService
{
List<IList<Answer>> GetAnswers();
}
interface IQuestionService
{
IList<Question> GetQuestions();
}
public interface ICalculationService
{
int CalculatePoints(Dictionary<Question, char> userAnswers);
}
Constants:
public static class Constants
{
public static class Answers
{
public const char A = 'a';
public const char B = 'b';
public const char C = 'c';
public const char D = 'd';
}
}
Core services:
static class Factory
{
public static T CreateInstance<T>() where T : new()
{
return new T();
}
}
Services:
public class AnswerService : IAnswerService
{
public List<IList<Answer>> GetAnswers()
{
return new List<IList<Answer>>() {
new List<Answer>() { new Answer(11, 3, "Sequoia"), new Answer(12, 3,
"Berch"), new Answer(13, 3, "Lindens"), new Answer(14, 3, "Alder") },
new List<Answer>() { new Answer(1, 1, "1"), new Answer(2, 1, "2"),
new Answer(3, 1, "5"), new Answer(4, 1, "6") },
new List<Answer>() { new Answer(7, 2, "More than 1"), new Answer(
8, 2, "More than 2"), new Answer(9, 2, "More than 5"),
new Answer(10, 2, "More than 6") },
new List<Answer>() { new Answer(15, 4, "yes, I do!"), new
Answer(16, 4, "Sure!"), new Answer(17, 4, "Exactly"), new
Answer(18, 4, "Yeap!") }
};
}
}
CalculationAnswerByAdding
class of Services:
class CalculationAnswerByAdding : ICalculationService
{
public int CalculatePoints(Dictionary<Question, char> userAnswers)
{
var sum = 0;
foreach (var question in userAnswers)
{
if (question.Key.Answer == question.Value)
sum += 1;
}
return sum;
}
}
CalculationAnswerBySubtracting
of Services:
class CalculationAnswerBySubtracting : ICalculationService
{
public int CalculatePoints(Dictionary<Question, char> userAnswers)
{
var sum = 10;
foreach (var question in userAnswers)
{
if (question.Key.Answer == question.Value)
sum -= 1;
}
return sum;
}
}
public class QuestionService : IQuestionService
{
public IList<Question> GetQuestions()
{
return new List<Question>() {
new Question(1, "How many are there contintents?",
Constants.Constants.Answers.A),
new Question(2, "How many are there colours?", Constants.Constants.Answers.B),
new Question(3, "What is the tallest tree?", Constants.Constants.Answers.C),
new Question(4, "Do you like dolphins?", Constants.Constants.Answers.D),
};
}
}
And Program.cs:
Main()
method of Program.cs
:
static void Main(string args)
{
IQuestionService questionService = Factory.CreateInstance<QuestionService>();
var questions = questionService.GetQuestions();
IAnswerService answerService = Factory.CreateInstance<AnswerService>();
var answers = answerService.GetAnswers();
var questionAnswers = questions.ToDictionary(q => q,
q => answers
.SelectMany(a => a)
.Where(b => b.QuestionId == q.Id)
.ToList());
var userAnswers = new Dictionary<Question, char>();
GetAsnwers(questionAnswers, userAnswers);
ICalculationService calculationService = Factory
.CreateInstance<CalculationAnswerByAdding>();
var userSum = calculationService.CalculatePoints(userAnswers);
Console.WriteLine(userSum > 3 ? $"Yeah, it is great. Your points are {userSum}."
: $"Hey, it is great. Your points are {userSum}");
}
GetAsnwers()
method of Program.cs
:
private static void GetAsnwers(Dictionary<Question, List<Answer>>
questionAnswers, Dictionary<Question, char> userAnswers )
{
foreach (var questionAnsw in questionAnswers)
{
AskQuestion(questionAnsw);
List<char> allowedAnswers = new List<char>()
{
Constants.Constants.Answers.A,
Constants.Constants.Answers.B,
Constants.Constants.Answers.C,
Constants.Constants.Answers.D,
};
while (true)
{
var userKey = Console.ReadKey().KeyChar;
Console.WriteLine();
if (!allowedAnswers.Contains(userKey))
{
AskQuestion(questionAnsw, true);
}
else
{
userAnswers.Add(questionAnsw.Key, userKey);
break;
}
}
}
}
AskQuestion()
method of Program.cs
:
private static void AskQuestion(KeyValuePair<Question, List<Answer>> questionAnswer,
bool showPossibleKeys = false)
{
if (showPossibleKeys) {
Console.WriteLine();
Console.WriteLine("Possible keys are A, B, C or D");
}
Console.WriteLine(questionAnswer.Key.Content);
questionAnswer.Value
.ForEach(a => Console.WriteLine(a.Content));
}
Guys, please, see my code. Is my code solid? Do I correctly use strategy and factory patterns? Any improvements and comments are greatly appreciated.
In addition, do my classes organized well? Especially, is it okay that I moved Factory
into folder CoreServices
? Or is there a better way to organize classes?
c#
I have a simple quiz game where user can give simple answers to simple questions and program will give a result.
Model classes looks like that:
public class Answer
{
public Answer(int id, int questionId, string content)
{
Id = id;
Content = content;
QuestionId = questionId;
}
public int Id { get; private set; }
public int QuestionId { get; private set; }
public string Content { get; private set; }
}
public class Question
{
public Question(int id, string content, char answer)
{
Id = id;
Content = content;
Answer = answer;
}
public int Id { get; private set; }
public string Content { get; private set; }
public char Answer { get; private set; }
}
Abstractions:
interface IAnswerService
{
List<IList<Answer>> GetAnswers();
}
interface IQuestionService
{
IList<Question> GetQuestions();
}
public interface ICalculationService
{
int CalculatePoints(Dictionary<Question, char> userAnswers);
}
Constants:
public static class Constants
{
public static class Answers
{
public const char A = 'a';
public const char B = 'b';
public const char C = 'c';
public const char D = 'd';
}
}
Core services:
static class Factory
{
public static T CreateInstance<T>() where T : new()
{
return new T();
}
}
Services:
public class AnswerService : IAnswerService
{
public List<IList<Answer>> GetAnswers()
{
return new List<IList<Answer>>() {
new List<Answer>() { new Answer(11, 3, "Sequoia"), new Answer(12, 3,
"Berch"), new Answer(13, 3, "Lindens"), new Answer(14, 3, "Alder") },
new List<Answer>() { new Answer(1, 1, "1"), new Answer(2, 1, "2"),
new Answer(3, 1, "5"), new Answer(4, 1, "6") },
new List<Answer>() { new Answer(7, 2, "More than 1"), new Answer(
8, 2, "More than 2"), new Answer(9, 2, "More than 5"),
new Answer(10, 2, "More than 6") },
new List<Answer>() { new Answer(15, 4, "yes, I do!"), new
Answer(16, 4, "Sure!"), new Answer(17, 4, "Exactly"), new
Answer(18, 4, "Yeap!") }
};
}
}
CalculationAnswerByAdding
class of Services:
class CalculationAnswerByAdding : ICalculationService
{
public int CalculatePoints(Dictionary<Question, char> userAnswers)
{
var sum = 0;
foreach (var question in userAnswers)
{
if (question.Key.Answer == question.Value)
sum += 1;
}
return sum;
}
}
CalculationAnswerBySubtracting
of Services:
class CalculationAnswerBySubtracting : ICalculationService
{
public int CalculatePoints(Dictionary<Question, char> userAnswers)
{
var sum = 10;
foreach (var question in userAnswers)
{
if (question.Key.Answer == question.Value)
sum -= 1;
}
return sum;
}
}
public class QuestionService : IQuestionService
{
public IList<Question> GetQuestions()
{
return new List<Question>() {
new Question(1, "How many are there contintents?",
Constants.Constants.Answers.A),
new Question(2, "How many are there colours?", Constants.Constants.Answers.B),
new Question(3, "What is the tallest tree?", Constants.Constants.Answers.C),
new Question(4, "Do you like dolphins?", Constants.Constants.Answers.D),
};
}
}
And Program.cs:
Main()
method of Program.cs
:
static void Main(string args)
{
IQuestionService questionService = Factory.CreateInstance<QuestionService>();
var questions = questionService.GetQuestions();
IAnswerService answerService = Factory.CreateInstance<AnswerService>();
var answers = answerService.GetAnswers();
var questionAnswers = questions.ToDictionary(q => q,
q => answers
.SelectMany(a => a)
.Where(b => b.QuestionId == q.Id)
.ToList());
var userAnswers = new Dictionary<Question, char>();
GetAsnwers(questionAnswers, userAnswers);
ICalculationService calculationService = Factory
.CreateInstance<CalculationAnswerByAdding>();
var userSum = calculationService.CalculatePoints(userAnswers);
Console.WriteLine(userSum > 3 ? $"Yeah, it is great. Your points are {userSum}."
: $"Hey, it is great. Your points are {userSum}");
}
GetAsnwers()
method of Program.cs
:
private static void GetAsnwers(Dictionary<Question, List<Answer>>
questionAnswers, Dictionary<Question, char> userAnswers )
{
foreach (var questionAnsw in questionAnswers)
{
AskQuestion(questionAnsw);
List<char> allowedAnswers = new List<char>()
{
Constants.Constants.Answers.A,
Constants.Constants.Answers.B,
Constants.Constants.Answers.C,
Constants.Constants.Answers.D,
};
while (true)
{
var userKey = Console.ReadKey().KeyChar;
Console.WriteLine();
if (!allowedAnswers.Contains(userKey))
{
AskQuestion(questionAnsw, true);
}
else
{
userAnswers.Add(questionAnsw.Key, userKey);
break;
}
}
}
}
AskQuestion()
method of Program.cs
:
private static void AskQuestion(KeyValuePair<Question, List<Answer>> questionAnswer,
bool showPossibleKeys = false)
{
if (showPossibleKeys) {
Console.WriteLine();
Console.WriteLine("Possible keys are A, B, C or D");
}
Console.WriteLine(questionAnswer.Key.Content);
questionAnswer.Value
.ForEach(a => Console.WriteLine(a.Content));
}
Guys, please, see my code. Is my code solid? Do I correctly use strategy and factory patterns? Any improvements and comments are greatly appreciated.
In addition, do my classes organized well? Especially, is it okay that I moved Factory
into folder CoreServices
? Or is there a better way to organize classes?
c#
c#
asked 6 mins ago
StepUp
237310
237310
add a comment |
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f210472%2fsimple-quiz-game-with-strategy-and-factory-pattern%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f210472%2fsimple-quiz-game-with-strategy-and-factory-pattern%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0nfhIBWq1tmO KygF